1. Introduction and Goals
This project is focused on developing an application to manage routes using SOLID specifications. The main objective is to ensure that users have control over their route data. For the realization of this application we will use the React framework.
1.1. Requirements Overview
Functional
-
Users can see the routes on a map.
-
Photos and videos may be shared through the application with other friends.
-
Users will be able to have the data stored in their own pod
-
Users may have groups of friends to share their routes.
-
The application must be able to load routes generated with a different application.
No functional
-
The application must have a friendly interface.
-
Ease of use by non-technical people.
-
Responsiveness of the application must be good.
1.2. Quality Goals
Priority |
Goal |
Description |
1 |
Security |
Give users ownership of their information and grant them control over access to it |
2 |
Usability |
Create a friendly interface that does not require prior knowledge |
3 |
Interoperability |
The application must be compatible with other applications. |
4 |
Compatibility |
Support different types of route formats and images. |
1.3. Stakeholders
Role / Name |
Contact |
Expectations |
Users |
Use the application to save and check your routes |
They hope that the application is easy to use, functional and safe. |
Teachers |
Supervise and evaluate the project |
Expect students to perform a functional extension complying with the requirements. |
Team |
Responsible for developing the app |
They hope to make a good application and learn to use new technologies. |
Inrupt |
Company that will test and evaluate the app |
They expect to get an application that uses SOLID |
2. Architecture Constraints
3. System Scope and Context
In this part we talk about the communication in two contexts, one is business context where we specifies communication partners with explanations of the domain and some schemes and the other one is the technical context where we do the same in a technical context.
3.1. Business Context
In this part we use an scheme to specify the communications of the person who is using the application with the application. First of all he logins with Solid, then when he is inside the appication we have to commuicate with the pods to store or see routes, if we want to send any route we have to communicate with the other pods of the users.
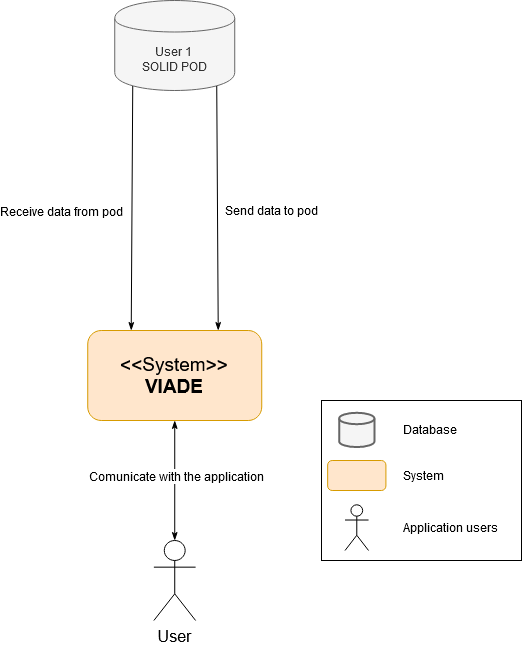
Object |
Description |
User |
Communicate with the application |
Viade |
Is the application itself. |
Solid Pod |
It will contain all the data from the given user. |
3.2. Technical Context
In this part we have to specify the same we had in previous point but with a technical view.
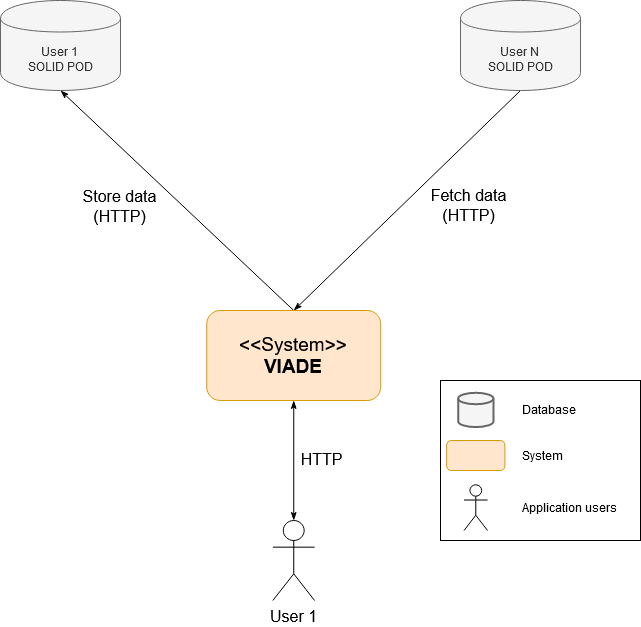
Object |
Description |
User |
Communicate with the application |
User POD |
Represents the communication will be made using internet with the HTTP protocol. |
Pod |
This is a Solid Pod and it will contain all the data from the given user |
SOLID |
Is the server in which the Solid pods are stored |
4. Solution Strategy
Viade_es3b is a decentralized application using SOLID, it was created with the entity ruta which is the most important of all aplication to load routes and store in SOLID PODs. To load and store the information, it uses libraries to load and store information in SOLID PODs with Javascript language.
We used Arc42 to define the structure of the documentation and we use Asciidoctor to deploy it into an HTML to see it.
We used React to build the front-end of the application because the teacher told us to use it and is the first step to do the application more usable, which is one of the quality attribute we wanted to perform in the application.
The interoperability of the application will be achieved using Json and load this kind of files in the POD. To achieve this objective we will follow the standard defined in https://github.com/Arquisoft/viadeSpec.
Regarding testeability: We tried to implement tests in the application because being testeable was one of the quality attributes, we di an application with several tests makeing it testeable in high level.
We deploy the application using github pages to increment the performance, the efficiency of the application is based on javascript calls to the Solid POD, so we tried to do the bes in this aspect.
5. Building Block View
In this section we show the static decomposition of the system into building blocks as well as their dependencies by means of a hierarchial collection of black boxes and white boxes and their descriptions.
5.1. Scope & Context
White box description of the overall system together with black box descriptions of all contained building blocks. See System Scope and Context
5.2. Level 1
At this early stage of the development process, this diagram contains as much detail as we can represent about the current view that we, the development team, have.
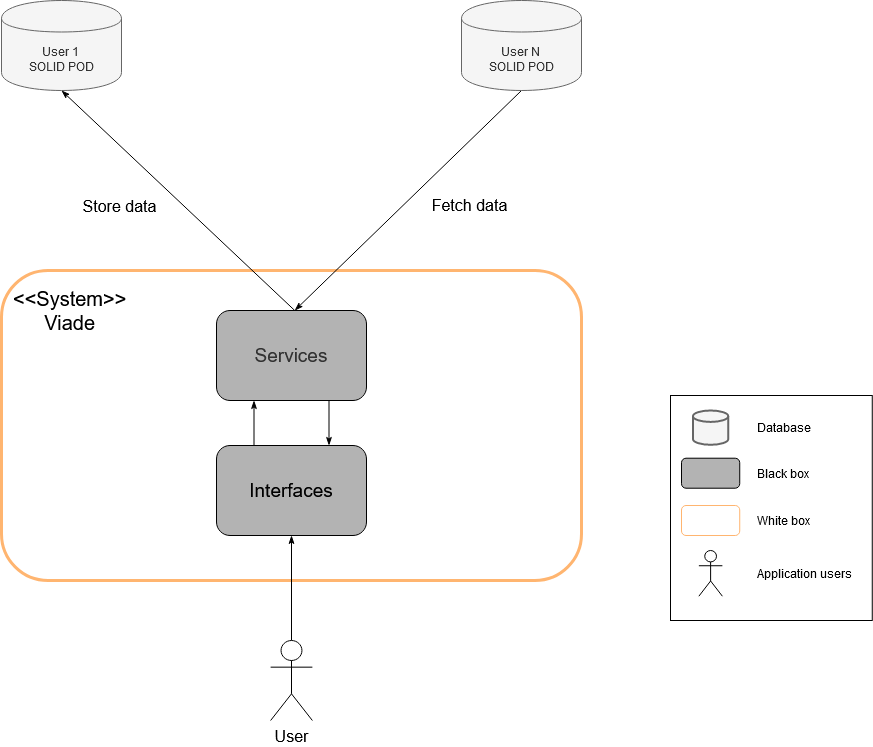
Object |
Description |
Solid Pod |
It will contain all the data from the given user |
Services |
Provides logic to perform actions |
Interfaces |
Provides user interactions with the system |
5.3. Level 2
In this view, we have a representation of the components of the system from a standpoint of the technologies used to implement each module.
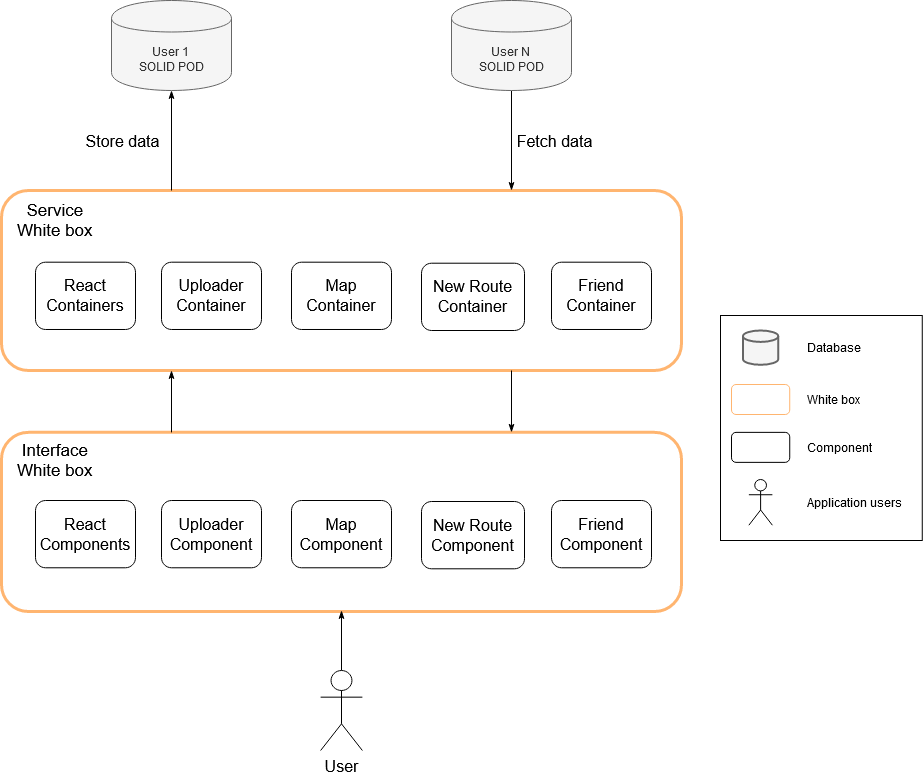
Object |
Description |
React containers |
Login, register, uploader, profile… logic |
Uploader container |
Logic to upload routes, photos and videos |
Map container |
Logic to manage the leaflet Map |
New Route container |
Logic to create and store routes |
Friend container |
Logic to manage friends and get their solid name and public routes basic info |
React components |
Login, register, uploader, profile… interfaces |
Uploader component |
Interface to upload routes, photos and videos |
Map component |
Interface that displays private or shared routes |
New Route component |
Interface to create routes and upload photos and videos |
Friend component |
Interface to manage friends and get their solid name and public routes basic info |
This separations of the system’s parts was accomplished in order to give the development team a clearer view of how the information of the project’s subject was going to be spread, and also to allow them to focus on single areas of software technology to ease the soon to come implementation task.
6. Runtime View
On this section, we will be talking about different scenarios a final user can see on the application
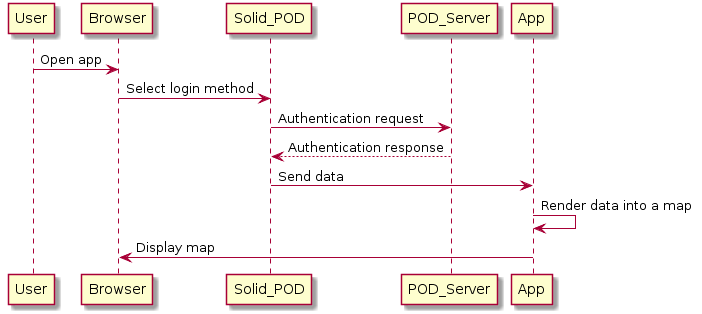
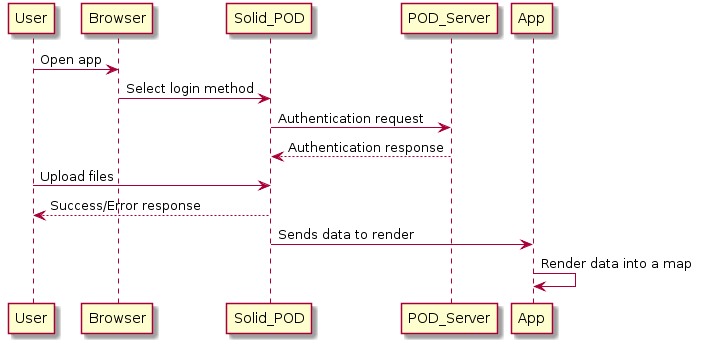
7. Deployment View
The application can be executed in any of the main browsers. For this you need a computer with internet connection and a SOLID POD, where the route data is stored. In this way, you can access to the application from anywhere, as long as you have a computer and internet connection. Depending on the course in the development of the application, an attempt will be made to adapt the application for mobile devices.
7.1. Infrastructure Level 1
The infractructure of the application is formed by 3 nodes. The user is connected to the first node which is the computer of the user, the user opens his browser that is necessary to connect to the application. This browser which is the first node is connected to the Viade Application through GitHub Pages where is our application deployed. Then the application is connected to the last node which is formed by 2 things, the first represents our POD, and the second represents another POD. We have to communicate to our POD, the other POD or both to use the application. For the moment, this is what we have done and this is the infrastructure of the application. Our system only needs a computer with internet and a SOLID account, this is the infractrusture possible for all the scenarios, if we do something like adapt it to mobile devices we can add another infrastructure.
8. Cross-cutting Concepts
This section describes overall, principal regulations and solution ideas that are relevant in multiple parts of the system.
8.1. Domain Model
8.1.1. Route
In the application , the base object we use and we modify is the object route. In the application we create objects route and we communicate this object with the user and POD to store and retrieve information of it. Each route has a name,description,media,points and a comment atribute which store this in the application.
8.1.2. User
The user is the person who uses the application and can post routes and share routes with another user.
8.2. Architecture and desing patterns
We followed a P2P architecture because is the standard architecture of an application like this we implemented. There are only patterns in the creation of the objects of the application, we used prototype pattern to create those objects.
8.3. User Experience
8.3.1. User Interface
The user interface is developed with React, we tried to do it easy to use and easy to understand to all kind of people.
8.3.2. Internationalization
The app is internationalized to be used in 2 languages, in English and Spanish for the people who can’t understand English. This makes the application more usable for all kind of people.
8.4. Development concepts
8.4.1. Building
To build the application we use Node.js and his package manager, we build the app with it and we use some of the features it has.
8.4.2. Testing
We have 3 types of tests in the application:
- TDD testing
-
Increment the code coverage and help us to improve the application. Performed using react-testing library.
- BDD testing
-
to check if the application works as it should. Performed using Cucumber and jest-puppeteer.
- Load testing
-
Simulation of multiple users accessing the application concurrently. Performed using Gatling.
8.5. Security and Safety
8.5.1. Security
The application security is provided by the Solid Authentication Client and the Solid POD, if you are not authenticated in the application, you can’t navigate in it.
8.5.2. Safety
No part of the system has an aspect that endangers the life of the user.
8.6. “Under-the-hood”
8.6.1. Persistency
The application is based on a decentralized system. Therefore, all information will be stored in each user’s personal POD. It will be the user who grants the read / write permissions.
8.6.2. Transaction Handling
The transactions realized across the application are asynchronous and we managed it to prevent unfinished transactions in the application to avoid errors.
8.6.3. Session Handling
Session is handled by Solid Authentication Client, so it need an account with a SOLID Pod created and it will be handled by it.
8.6.4. Exception/Error Handling
We tried to manage exception and error handling in the code of the application.
9. Design Decisions
This section will be to specify some of the design decisions we took to develop the project.
Decision |
Explanation |
Documentation |
Is done with asciidoctor, is open source and is usefull for us. |
Interface |
Built with React generator application and modified to do it more usable. |
Code language |
The language is Javascript. |
Architecture |
Peer-to-Peer because is the better in a Solid decentraliced app. |
Data store |
Solid Pods so we don’t need any database, the routes, the comments and the images are stored there. |
Map |
Leaflet is the library used to see the map in our applications because is open source and easy to use. |
Routes Format |
The format for our routes is GeoJSON because we used it in other subject and we are familiarizated with it. |
Testing |
There aretesting in the application to improve our code coverage and to improve it. |
Acceptance tests |
We developped some behaviour-driven tests via cucumber because we saw how it work in class and is easy to develop. |
10. Quality Requirements
10.1. Quality Tree
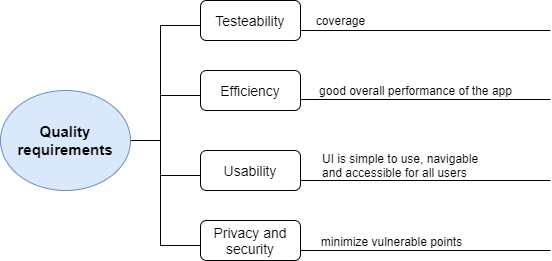
10.2. Quality Scenarios
Reference | Quality attribute | Scenario | Priority |
---|---|---|---|
1 |
Performance / efficiency |
The user will view the routes on a map as fast as possible. |
High, high |
2 |
Usability |
The system must be intuitive and easy to use for all types of users. |
High, medium |
3 |
Privacy and security |
Users can have private data that nobody can see if it does not allow it and the data will be separated from the application. |
High, medium |
4 |
Testeability |
The app should be testable, as automated as possible with the use of testing tools. |
Medium, medium |
11. Risks and Technical Debts
In this part of the documentation we will write about some risks and technical debts we will face in the development of this project.
Risk |
Explanation |
Technologies |
All the technologies we have to use in the application are news to us, so is a risk working with all of these applications and technologies. |
Teamates |
Working with so much people in a team without knowing each others is a risk in the development of the application. |
Time |
We have to do other subjects this semester, and this can become a problem for the development of this application. |
Language |
Using another lenguage in the applicaton is hard when you are not used to use it. |
Spec |
Is hard to negotiate with other teams or people for the standarization of the store of the rutes in the PODs. |
Load Time |
The load time of the application is elevated right now and we want to reduce it. |
Working with PODs |
Working with PODs and rdf is not easy for us, and we had some problems with it. |
12. Glossary
Term |
Explanation |
Solid |
is a web decentralization project led by Tim Berners-Lee, the inventor of the World Wide Web, developed collaboratively at the Massachusetts Institute of Technology (MIT). |
Leafletjs |
Leaflet is a widely used open source JavaScript library used to build web mapping applications. |
Javascript |
Is an interpreted programming language that conforms to the ECMAScript specification. JavaScript is high-level, often just-in-time compiled, and multi-paradigm. |
Arc42 |
Provides a template for documentation and communication of software and system architectures. |
Solid Pod |
Personal online data store . It allows users to save their information and the information they are sent. A POD can store photos, text, contacts or calendar events and many other things. |
React |
React is a JavaScript library for building user interfaces. It is maintained by Facebook and a community of individual developers and companies. |
Stakeholder |
Person with an interest or concern in something, in the area we are working on, the decentralized chat. |
Peer-to-Peer |
Peer-to-peer computing or networking is a distributed application architecture that partitions tasks or workloads between peers. Peers are equally privileged, equipotent participants in the application. They are said to form a peer-to-peer network of nodes. |
Rdf |
It has come to be used as a general method for conceptual description or modeling of information that is implemented in web resources, using a variety of syntax notations and data serialization formats. |
About arc42
arc42, the Template for documentation of software and system architecture.
By Dr. Gernot Starke, Dr. Peter Hruschka and contributors.
Template Revision: 7.0 EN (based on asciidoc), January 2017
© We acknowledge that this document uses material from the arc 42 architecture template, http://www.arc42.de. Created by Dr. Peter Hruschka & Dr. Gernot Starke.