1. Introduction and Goals
This project consists on developing an application that allows users to personalize a map of Brussels. In their map they can add the landmarks they want, like shops, sights or restaurants. In addition, users will be able to share their map information with the users they want while restricting other users from seeing it. Ideally, this application should easily be implemented for using it in a different city, while it should be interoperable with similar applications.
1.1. Requirements Overview
Users can:
-
Add landmarks to the map
-
Filter the information on the map
-
Share uploaded places with the desired users of the application
-
See other users' additions to the map
-
Add a review, comments or pictures to the uploaded places
-
Manage their data from their SOLID pods
1.2. Quality Goals
Goal |
Description |
Privacy |
The application must follow the SOLID project guidelines, by focusing on making sure that the users have control over their data |
Usability |
The application must be easy to use and intuitive |
Testability |
The application must be thoroughly tested to avoid unintended behaviour |
Interoperability |
The application must be compatible with the ones developed by oother students |
Performance |
The application must have quick response times |
1.3. Stakeholders
Role/Name | Contact | Expectations |
---|---|---|
Students / Developers |
Andrés Cadenas Blanco Jorge Joaquín Gancedo Fernández Pedro Limeres Granado Diego Villanueva Berros |
Develop a software we can be proud of while learning new technologies as SOLID |
HappySw |
HappySw |
An application that fulfils the contract agreed with the Council of Brussels |
Teacher |
José Emilio Labra Gayo |
Make sure the students follow the guidelines and progress correctly |
Council of Brussels |
Council of Brussels |
Have an application developed that meets their needs |
Users |
Whoever uses the application |
A functional and easy to use application |
2. Architecture Constraints
During the development of this LoMap project, there are some constraints that must be taken into account. These constraints are the base of the architecture process, and are the roots of the final development. They are summarized and briefly explained in the following table.
Constraint | Description |
---|---|
SOLID |
Provided by the stakeholders. A way of building decentralized social apps, it gives every user a choice about where data is stored, helping the privacy of each user. |
Github |
The development team was given a starting draft of a project in GitHub as a public repository. From then on, all the work related with this project will be tracked and uploaded in this repository. |
Time |
The application is developed in a semester during a course, that means time is limited. |
3. System Scope and Context
Lomap is a web application whose objective is to have markers and routes saved in a map where the user has total control of their data. They can also add and share this information with other "friends" sharing the platform. In order to achieve the control of their data SOLID technologies are used all along the project. A user will be able to post an dplace markers, they will also have the capability of viewing the ones of their online "friends".
There is clearly 5 different actors regarding business context, however one is the SOLID pod of both admin and user actor. Which is "integrated" in them making it be a "subsystem". As a result we obtain the following use case scenario.
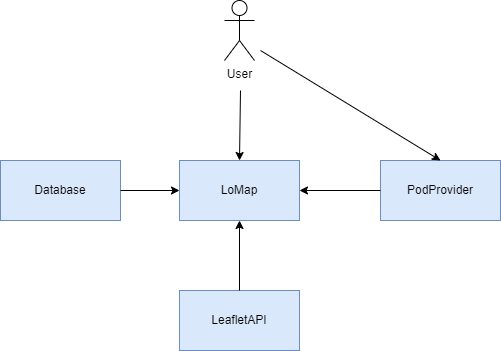
From that we can say that the main actors have this speciofications:
User |
This type of user is the "average" user as it has an account and it is allowed to create routes or markers, following people and viewing different maps. They connect to the webapp using the pod provider |
Pod Provider |
A pod provider using SOLID architecture where all the sensitive data of the users will be stored. |
lomap |
The web service itself. Here the user is able to access and modify their maps same as looking the other’s. |
database |
This will be a database in charge of containing the minimal data to make the web application to work and for it not to be heavily slowed down by the decentralized approach. |
leaflet |
Leaflet API is an api using openmaps which is simple and easy to develop in it to create personalizzed maps. |
4. Solution Strategy
In this section we are explaining the main decisions we took in the development of the project. Up until now, we have made some decisions about the project, those decisions can be organized in different categories:
-
Technology decisions:
-
React: JS framework that helps with the user interface of the web application.
-
Map API: Used to plot the map in the application in order to allow the user to do every possible action. The selected API to do it is Leaflet.
-
-
Decisions on top-level decomposition:
-
N-Layers: We are using an N-Layers pattern of two components: the controller and the frontend. The former can be found in /restapi/ and the latter in /webapp/.
-
Editor: We are all using Visual Studio Code to work on this project, it is the editor we are used to program in and it has some features that made us work using it.
-
Github: The project is on a public repository as given by the professors of the subject. We are all able to work using it, and the main features of it are the pull requests in order to upload code.
-
-
Decisions on quality goals:
-
Usability: Our map application is quite easy to use for everyone, we are focusing on the ease of the user interaction and comprehension.
-
Security: It is the main point of our application, as we are using solid, we are maintaining the privacy of every user who logs into LoMap.
-
Response times: As much as possible, we are trying to minimize response times of the application in order to be more user friendly.
-
-
Organizational decisions: During the development of this project, everyone is the group is in constant contact with the rest of the members of the team, mainly in the weekly meeting which takes part during lab classes throughout the semester. In addition to that, we used a whatsapp group in order to consult with each other little things that could emerge during the week. Related with the project, our main way of working with the code was through pull requests. Each one of the members did some work, in his newly created branch and when he ended his work, he committed the changes and requested a pull request in GitHub, where another person in the group should revise the changes and aprove that pull request in order to merge the changes to the main branch. In order to do advances in the development we are using the issues in Github as well, in that way we make sure that we do everything we consider necessary. At last, we are using the wiki on Github as well, for creating minutes of every meeting the team does, for saving the main topics treated on each one, the main decisions taken, the participants that took part, and some other important things in order to keep track of the progress made.
5. Building Block View
5.1. Level 1 Diagram
- Motivation
-
This diagram is motivated by the need of having a clear separation of the composition of the application.
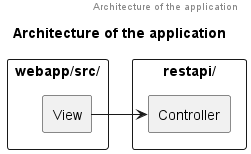
- Contained Building Blocks
-
As seen, the different components are the following:
-
View: The view layer is composed of those elements that the client will interact with; it includes the React code, CSS sheets and the client-side code.
-
Controller: The controller layer will ensure a proper communication the client and the server. It currently handles also persistence.
-
5.1.1. View layer
Purpose/responsability
As already explained this layer will be composed of those components that the user will interact with.
Interfaces
Since we are not using interfaces, we will be seeing important components:
-
The main UI: It is divided in several components: the left bar, the navbar and the rightbar, each one with its one subfolder in /webapp/src/components.
-
The pages: These are the React components that compose the web. They can be found in /webapp/src/pages, in their own folder.
Open Issues/Problems/Risks
-
The functionality to see friends is yet to be implemented. This one issue is transversal to the application.
5.1.2. Controller layer
Purpose/responsability
The controller layer will handle internal communication between the view and the rest of the application.
Interfaces
-
The controllers: they handle the logic and the persistence of the application. They can be found in the restapi folder.
Open Issues/Problems/Risks
No issues, problems or risks are known.
6. Runtime View
The following sequence diagrams describe different situations that happen during the use of LoMap. These situations where chosen as they show the impact of our architectural decissions.
6.1. Accessing the application
This diagram shows the communication between the user, the app and the pod when the user logs in.
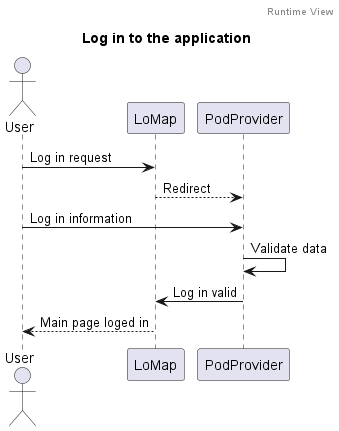
6.2. Adding landmark to the map
This diagram show the communication between the user, the app and the pod when the user adds a landmark.
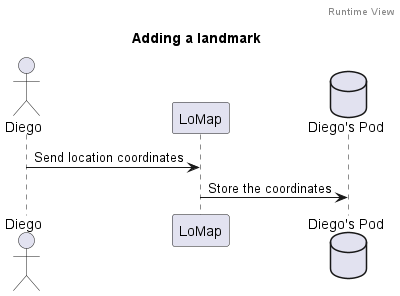
6.3. Seeing friends' added landmarks
This diagram show the communication between the user, the app and the pods when the user wants to see their friends' landmarks.
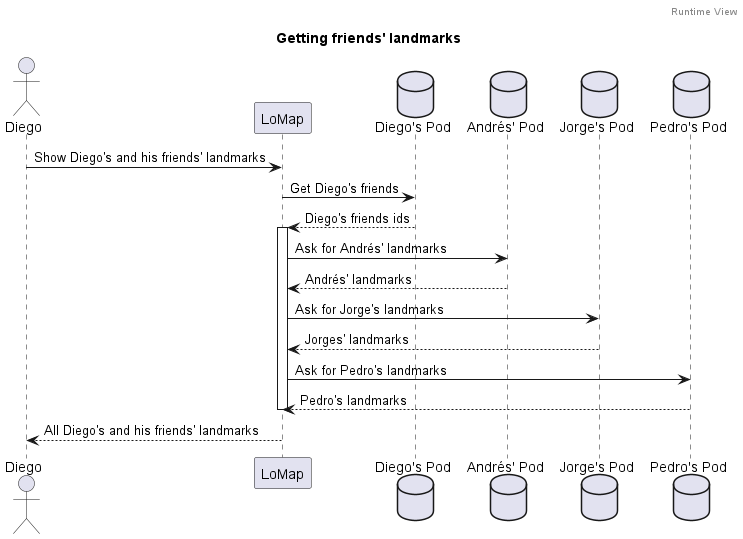
7. Deployment View
7.1. Infrastructure Level 1
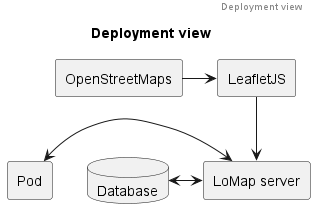
- Motivation
-
This approach is motivated because it is not very complicated, and can be easily changed at later date if necessary. It also removes the dependency in the same machine of the database and the server, so if one is down, we can launch it in another system so long we change the machine hosting one service or the other. We are also depending on an external provider, that being OpenStreetMaps, to supply the map we need for the application to work, so the will run so long OpenStreetMaps does work.
- Quality and/or Performance Features
-
This approach has a series of upsides and downsides.
On one hand, its simplicity means it can be deployed easily changed without much changes at a later dater. On the other hand, this deployment scheme depends on both servers not being down, and if either or OpenStreetMaps is down, then the application wouldn’t properly work. Also, we have to consider that users might have different pod providers (and as much as we’d like to, it simply is not feasible to test the application in every single pod provider), so there’s another possible bottleneck there.
- Mapping of Building Blocks to Infrastructure
-
As it can be seen, this approach makes the pods be connected to the platform at nearly all times. Currently, we have discussed that the database will store fixed data that all users will necessarily retrieve, but that may change in the future, as a common data schema has yet to be defined with the rest of the implementations.
7.2. Infrastructure Level 2
7.2.1. The server
Although we have yet to choose the operating system that will run it, it will probably run on Linux. On the one hand, using Linux means we do not have a graphical interface but if we consider necessary we can install.
7.2.2. The database management system
We have chosen to use MongoDB. This decision was considered due to the experience of one member of the group using MongoDB through Mongoose, as well as using Typescript to run the product and, thus being posible to manipulate the data directly using JSON notation.
8. Cross-cutting Concepts
Some cross-cutting concepts of the system will be detailed here. These can give a broader look of our application
8.1. Domain model
Users can have several friends as well as having added several reviews or landmarks. In addition, landmarks will have reviews associated to them and viceversa.
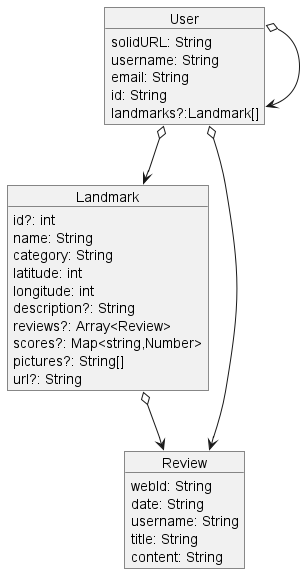
8.2. Security
This is probably the most important concept of our application. To give users the most safety we only store the information necessary to function and we will store most of it in users' SOLID pods, this means that users will have power over how, where and with who they share their data.
8.3. User experience
We want to focus on creating an app that is easy to use and intuitive. Thus, we will follow the global standards so users know what to do without thinking too much. Some of these are having menus on a side, using similar colors as other map applications…
8.4. Architectural and design patterns
The application will be based on the MVC architectural style using a N-layer structure. This will make the code clean, easy to change and organised.
9. Design Decisions
Decision | Explanation |
---|---|
React |
Taken as a recomendation by some teachers. In addition, one of the members of the group has already worked with it in the past. It allows us to build a good user interface for the application. |
Leaflet |
It is the provider we are going to use for obtaining the necessary features around the map. We studied some other different options, such as Mapbox o Google Maps API, but we needed the Api to be completely free. |
Solid Pods Providers |
We are trying to allow the user to connect to the application with any solid pod provider. |
React Libraries |
In order to make our life easier while the development of the application, we made the decision to include some libraries in the project. These libraries we used are listed on issue #42. |
SonarCloud |
This has been presented to us on one laboratory, it measures how many lines of code are being tested using TDD. |
Cucumber |
In order to make the mandatory BDD tests, we are going to use a tool that we somewhat knew from other courses in the degree, used to make the acceptance tests. |
Prioritize |
As we have seen until the third deliverable, we are having some trouble on some things. As a result, we have decided to focus on the more functional requirements on this last deliverable. |
MongoDB |
The application main place for storing information is still Solid. We made the decision to store in a database on the cloud with the users that log into our app. Each time a new user logs into the lomap application, its username and webID of solid are stored in our database in order to help the interactions of users on the app, making sure each user can only see the friends that have logged into the app. |
Landmarks |
We have been dealing with a problem related with the persistence of the landmarks on solid, as a temporal solution, we decided to store them in the mongo database, which in the future will be changed. |
Friends |
We had a little discussion over this topic on a meeting, reaching an agreement over only allowing the users to add friends via lomap. As the way of adding friends is quite special on this app and is done via Solid, we decided not allowing to cancel a friendship via lomap, as we thought it was something external to lomap, more related only with Solid. |
Coordinates |
When we were dealing with adding landmarks, we were trying to add coordinates by writing the number, and we are changing our inicial approach to be able to click on the map and retrieve the coords of that point. |
The old map interface |
In prior versions, we used a custom Map component to handle the map logic. This component has been discarded in version 1.1 due to it becoming an obstacle when implementing event handling related to the map. |
Monitoring and profiling |
We are quite short of time so we decided to try to improve the tests and the features of the app rather than recording our perfonmance, as it was optional, we thought we should put all our focus on delivering a nice app. |
Puppeteer |
We think it was the easier way in order to implement the necesary e2e tests for the application, we considered other options seen in class and that we tried searching for, but finally decided to use puppeteer. |
Use of pods and interoperability |
We as a team participated in the debates on the other github issues about the interoperability, not arriving to a very clear conclusion. As a result we spoke to some colleagues in order to try and make our applications interoperable, causing this decision to change our approach of storing landmarks on the pods. |
Way of storing landmarks |
Connecting with the previous decision and probably as a result of having trouble with storing information on solid through the restApi, we decided to stop that approach and start dealing with writing and reading from the pods from the webApp. |
Tests |
On the lasts days we arrived quite short of time and we could do everything we were requested but not all tests run correctly, this is not due our application, because obviously we’ve tested everything via executing our app, but the setup of some tests of the restApi may not be working properly. |
e2e Tests |
We have been dealing with a problem on these tests almost for 3 weeks before the final deliverable. The tests start executing perfectly and the login is done as it should, but when the home page must load, the page is not loaded in a redirect, that outside testing works perfectly, for some reason we did not realised. We decided to keep the code related with the tests as we think it has to be ok, although the test do not succeed. |
Structure of the app |
We decided to keep a leftBar in order to navigate through all the pages of our application, as a result of this decision, we needed to place different maps in different pages of the app, as we don’t always keep the same instance of the map. |
Structure of the presentation |
We have decided to focus on different things in the presentation on the final day, we will explain some of the problems we had, as well as revising the main structure of our application and making a draft on how to navigate on our app via showing how we use it. Just in case the day of the presentation the app does not work, we are preparing a short video of how it can be used too. |
10. Quality Requirements
10.1. Quality Tree
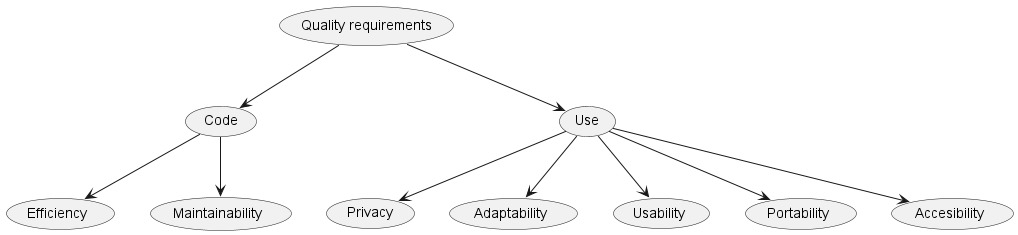
10.2. Quality Scenarios
Part |
Name |
Priority |
Description |
Code |
Maintainability |
High |
The code written must be maintainable, and must be easy to work with it. |
Efficiency |
High |
The code must be as efficient as possible, without discarding security (see next row) |
|
Use |
Adaptability |
Medium |
As it will have multiple users, the final result must be adaptable to different screen sizes. It is worth noticing that this may not be the case at all with mobile phones, as the screen size is usually significantly smaller. |
Accesibility |
Low to Medium |
From a realistic point of view, a map is by definition non-accesible to blind people. Thus, we will limit accesibility to daltonic people. |
|
Privacy |
High |
The final product must adhere to the SOLID principles, being therefore private. |
|
Usability |
High |
The final product must be usable by users. |
|
Portability |
High |
The final product must be interoperable with other similar products. |
11. Risks and Technical Debts
As it stands now, we have identified some important risks before starting coding the project.
Risk | Mitigation proposed | Consideration |
---|---|---|
Use of technologies we are not used to. |
Consulting the documentation of the languages when necessary, as well as trying to use technologies we know, so as to better concentrate on those we do not. Helping ourselves is also certainly a solution. |
High |
Github use. |
We are all familiar with how to use both git and GitHub, so we do not necessarily consider this as a risk. Nevertheless, it important to remember that working on not-updated branches can have severe consequences on the future, so we must remember to perform frequent commits and atomic branches: branches for a single feature or update. |
Low |
SOLID |
Inform ourselves about SOLID, as well as trying to talk about it ourselves to see if there is anything we have missed. Asking the professor when arriving at a doubt. |
High |
Team members leaving the team during development. |
Assign a feature to two people instead of one, mitigating (though not avoiding) the consequences of a person leaving mid-development. Doing proper documentation in the code and commit messages should also help. |
Medium |
Regarding the technical debt, it is probably going to be very high, because we won’t be using a single technology we are familiar with (except one member). Both SOLID and React are probably the most difficult parts, and we are going to have huge amounts of technical debt on both of them. Currently, we do not know any mitigation system other than searching possible solutions in Internet.
12. Glossary
Term | Definition |
---|---|
N-Tier architecture |
Client–server architecture in which different layers are separated allowing the development of flexible and reusable applications. |
SOLID |
SOLID is a web decentralization project where the users have control over their data. It is led by the inventor of the WWW, Tim Berners-Lee. SOLID guarantees that users decide what and with whom they should share their data. |
POD |
Secure personal web servers for data. When data is stored in someone’s Pod, they control which people and applications can access it. |
About arc42
arc42, the Template for documentation of software and system architecture.
By Dr. Gernot Starke, Dr. Peter Hruschka and contributors.
Template Revision: 7.0 EN (based on asciidoc), January 2017
© We acknowledge that this document uses material from the arc 42 architecture template, http://www.arc42.de. Created by Dr. Peter Hruschka & Dr. Gernot Starke.