1. Introduccion and goals
We intend to develop an online store using SOLID and REACT technologies. The store will allow the user to purchase any of the available products, as well as to search through them and add them to a personal shopping cart.
1.1. General requirements
The website must mainly meet the following requirements:
-
The data storage shall be separated from the application itself.
-
The web will allow the user to search among the different products either explicitly or through text filters.
-
The system administrator will be able to add new products at any time as well as to consult the information of the clients.
-
Each user will be able to have his own personal basket which can only be consulted by the user in question and which will last in subsequent sessions.
-
Users will have the option to register by giving their personal data.
-
Only registered users will have access to a personal shopping cart.
-
All users will have the option to return any product just by providing the purchase code.
1.2. Quality goals
Given that those who will habitually use the web will be the users and the administrator, the main goals would be:
ID | Priority | Quality | Motivation |
---|---|---|---|
QG1 |
1 |
Privacy and Security |
All information regarding the data of users and their carts must be private. |
QG2 |
2 |
Usability |
The application must be intiutive for both administrator and users. |
QG3 |
3 |
Availability |
The application must be available all time for the users to buy products. |
QG4 |
4 |
Performance |
Response times should be as short as possible so as not to make the user impatient with a low latency. |
1.3. Stakeholders
The stakeholders will be the developers, the users and the teachers.
Role | Expectations |
---|---|
Developers |
Work with the architecture and code as well as the documentation and help in decision making. |
Teachers |
Overseeing the project |
Clients |
Users who will be able to freely use the store either to make a purchase or a return or simply to consult the store. |
Administrator |
A special user who will be able to manage the products and client information from the app |
2. Architectural constraints
2.1. Technical constraints
Requirement | Explanation |
---|---|
React |
Framework oriented to the Frontend of the application. |
Typescript |
Programming language for business logic. |
Solid |
Solid Pods will be used to obtain customers' personal information. |
Hosting |
The application must be continuously accessible. |
Testing |
Automatic and manual tests on the code. |
2.2. Organizational constraints
Requirement | Explanation |
---|---|
GitHub |
GitHub provides tools such as issues and actions to help us in development. |
Working group |
The group is made up of 4 people. |
Meetings |
At least one team meeting per week will be held and recorded. |
Delivery times |
Approximately every 3 weeks there will be deliveries to know the status of the application and its documentation. |
2.3. Convections
Requirement | Explanation |
---|---|
Arc42 |
To develop the documentation we will follow the Arc42 model. |
SOLID |
SOLID principles will be followed with respect to customer privacy. |
3. System Scope and Context
The project we are going to develop is aimed at the online sale of technological products and video games.
These products will be grouped by sections within the page itself, and each product will have the following components:
-
Name.
-
Price.
-
Add to cart option.
-
View option (to show the details).
-
Image.
3.1. Business Context
The home page will show all the products available in the shop. The customer will be able to log into the service as well as to register himself, regardless of this he will be able to search for the desired technology by name or a price range, then the list of products corresponding to the selected filter will be displayed. If the usser is logged he will be able to add the products to a shopping basket, a list made up of all the products chosen by the customer to be purchased.
The customer will have access to his shopping basket where he will be able to increase or reduce each product quantity as well as remove them from the basket or finalise his purchase.
Entity | Inputs | Outputs |
---|---|---|
User |
Interface of the webapp |
Data that user provides to the application |
DeDe |
Interaction and data from the user and APIs |
Information to the users and data to the APIs |
MongoDB |
Data from DeDe to store |
Data required from DeDe to show to the users and admins |
PODs |
Inrupt username of the client |
Address of the client |
Cloudinary |
Image to store in |
ID of the image to show |
NodeMailer |
Email address of the client and details of the order |
Sends an email to the client |
Shippo |
Address of the client |
Shipping cost and estimated arrival day of the order |
3.2. Technical Context
The system has SOLID architecture in order to respect the privacy of each user’s data, this is achieved through the use of PODS to store the user’s personal data. The programming language used in the application is Typescript and React is used as the main library to facilitate the development of the application.A certain number of apis were also used to access certain resources.
Technology | Definition |
---|---|
SOLID |
Architecture to facilitate code cleanliness and maintainability |
PODS |
Personal data storage units |
TYPESCRIPT |
Language used for the development of the application |
REACT |
Main library to facilitate the development of the GUI |
CLOUDINARY |
API used to download and upload the product images and edit their properties |
NODEMAILER |
API which allows emails to be sent from different hosts (in our case Gmail). |
SHIPPO |
API which gives information about the shipping costs. |
4. Solution Strategy
4.1. Technologies
These are the technologies we have decided to use:
-
MongoDB: NoSQL database.
-
TypeScript: JavaScript superset, which mainly adds static typing to JavaScript.
-
GitHub: Allows to host Git-based projects.
-
SOLID: Project that aims to allow users to decide where their data is stored, in order to improve privacy.
-
Libraries
-
React: Library for creating user interfaces.
-
Node.js: Allows to execute TypeScript on the server side.
-
Express: Node.js web application infrastructure.
-
Mongoose: Library to connect to the database and add functionality.
-
Axios: Library to make async petitions.
-
Supertest and Jest: Modules to test our application.
-
jsPDF: Library to create PDFs, we will generate our invoices in PDF.
-
Programs:
-
Visual Studio Code: Code editor that thanks to its large number of extensions will facilitate our work.
-
Postman: Software to test APIs.
-
-
Deployment:
-
Amazon Web Services: Web server provider where we will deploy our application.
-
Docker: Project that automates deployment of applications inside containers. We will create containers for both restapi and webapp to deloy them on AWS.
-
-
APIs:
-
Cloudinary: Service that provides an API to upload and download the images of the products.
-
Nodemailer: API that allows us to send emails to the user with the details of the orders.
-
Shippo: Provides an API to calculate the shipping costs and the estimated day of arrival of the orders.
-
4.2. Architectural and design patterns
We have decided to follow the MVC (Model, View and Controller) pattern, which consists of separating the application data from the logic and the user interface.
4.3. Decisions to achive quality goals
In order to achieve the proposed objectives we have decided to distribute the work in such a way that part of the group focuses more on the backend and the other one on the frontend. We have taken this decision since all the technologies to be used are new for all of us, and we believe that it will be easier to achieve the objectives if we focus mostly on one part.
-
Having half of the group focused on frontend, we believe that it will be easier to to achive the usability goal.
-
To achive the availability goal, we will have the application deployed in Amazon Web Services and it will be accesible from everywhere.
-
Privacy and Security are another goals that we are focused on. To achive this, the users must have a username and password to access to the application, and we are going to use PODs to get the address from the user, so we are not storing any personal information apart from the name, email and DNI.
4.4. Organizational decisions
In order to work in an organized way and try to minimize errors, we have decided to organize ourselves as follows:
-
Creation of three automated dashboards (GitHub Projects), one for each part in which the project is divided.
-
Frontend
-
Backend
-
Documentation
-
-
Creation of several branches:
-
master: main branch of the project where stable versions of the project will be uploaded.
-
develop: development branch to which the changes made in both frontend and backend will be uploaded by pull request.
-
frontend: branch where frontend changes will be uploaded.
-
backend: branch where backend changes will be uploaded.
-
We will create branches to try things like deploying the application or adding risky features.
-
An individual branch for each developer to work on:
-
iker
-
pabloa
-
pablof
-
sergio
-
-
-
To communicate and make easier the organization we will use:
-
Microsoft Teams: We will meet weekly to make decisions.
-
WhatsApp: We will use it to make a quick comment, such as to warn of a sudden problem or things that come up.
-
GitHub: Here we will communicate through issues and the project board, where we will share tasks.
-
5. Building Block View
5.1. Whitebox Overall System
- Motivation
-
Overview DeDe and MongoDB structure.
- Contained Building Blocks
Name | Description |
---|---|
Actor |
Actor interacting with the application. |
DeDe |
Electronics sales application |
POD |
System that stores the user’s personal information |
MongoDB |
Database system to be used |
5.2. Level 2
- Motivation
-
Description showing how our application will communicate with the database and the users' PODS.
- Contained Building Blocks
Name | Description |
---|---|
User interface |
Part of the application that the user will see and interact with. |
Solid auth client |
Client with which we can manage the information of our users' PODS. |
Mongoose |
It will allow us to manage the database |
5.3. Level 3
- Contained Building Blocks
Name | Description |
---|---|
Webapp |
Part of the application that the user will see and interact with. |
Restapi |
Part of the application responsible for persistence. |
6. Runtime View
This section shows the main sequence diagrams between the users and the application.
6.1. Products catalog
This view shows a request from a customer to view the product catalog. The web receives the request where it will access the database to get the list of products. Once obtained, it returns them to the customer.
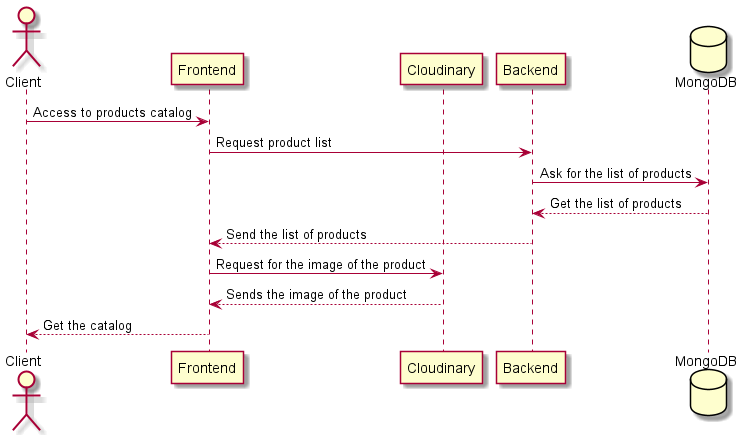
6.2. Login
This view shows the login process. The website receives the user’s credentials, and after a back-end petition the credentials will be verified and the user will be allowed to buy products.
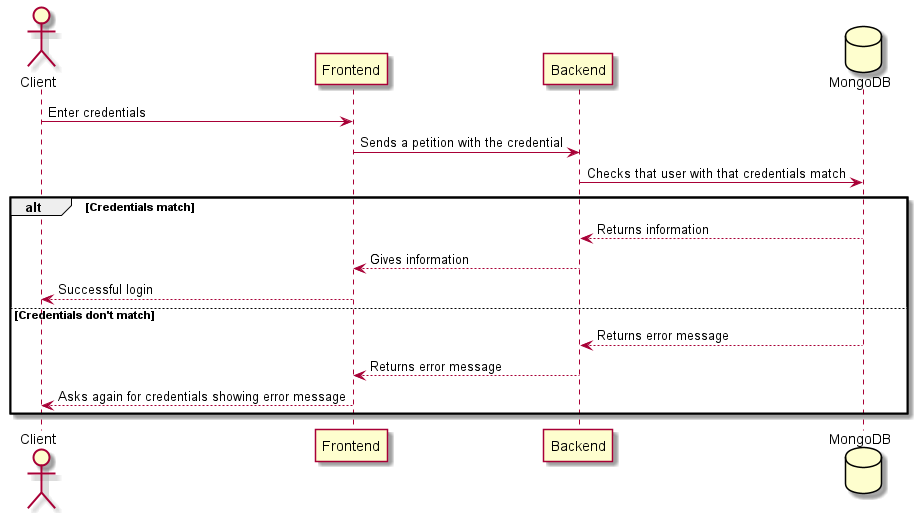
6.3. Buy process
This view shows a request from a customer to buy a product from the catalog.
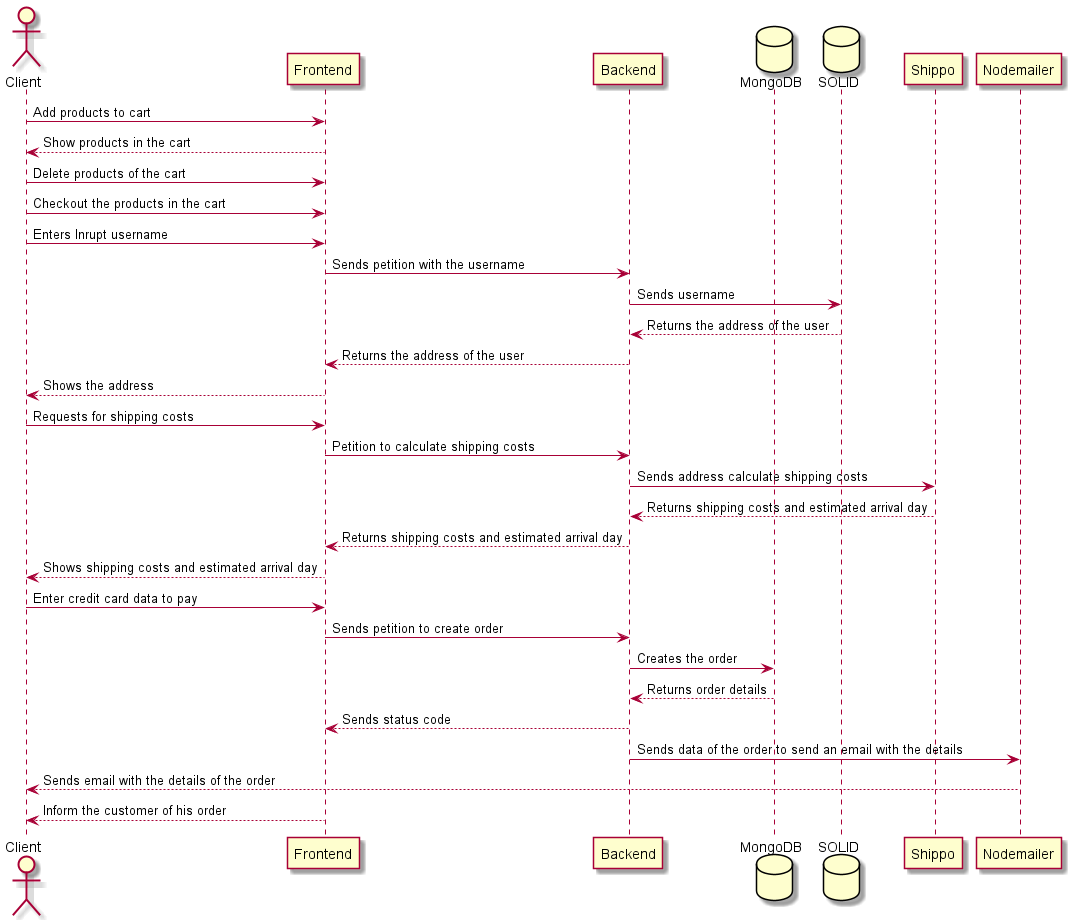
6.4. Sign up
This view shows the sign up process by a client.
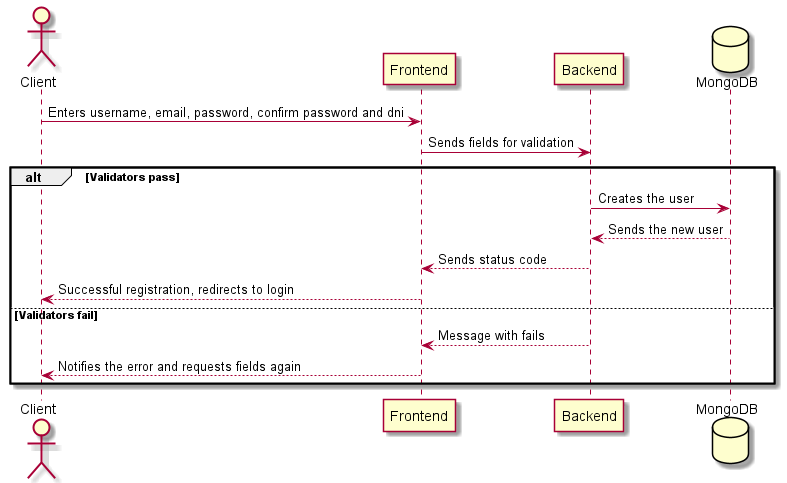
6.5. Modify products
This view shows how the administrator, after having logged in, will modify the catalog. The front-end makes a petition to the back-end by a petition and then the app connects to the database.
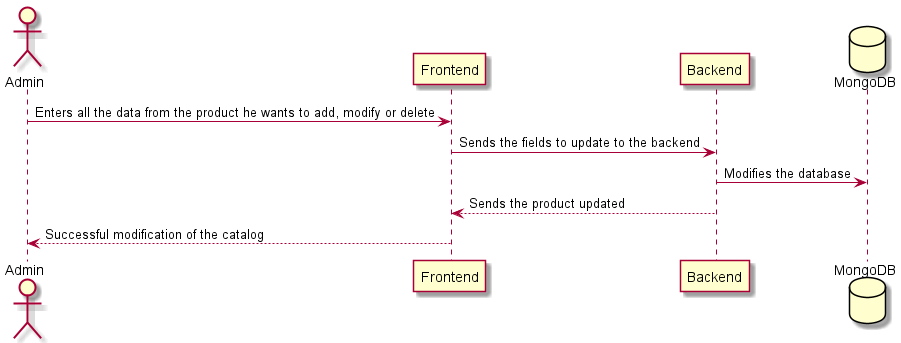
6.6. View orders
This view shows how a client will obtain a list of all his orders.
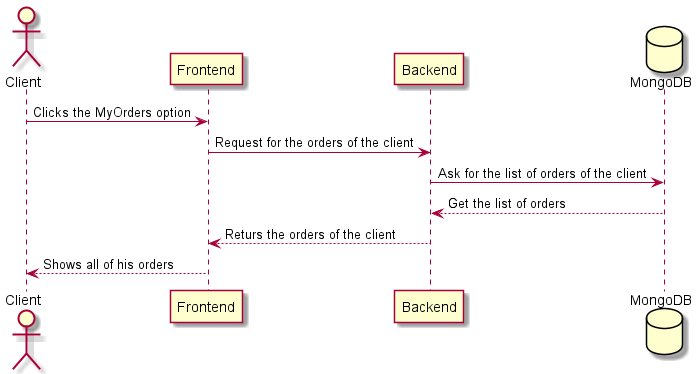
7. Deployment View
- Necessary infrastructure
-
To use our online shop you only need a mobile device (mobile phone, tablet…) or a computer and Internet connection.
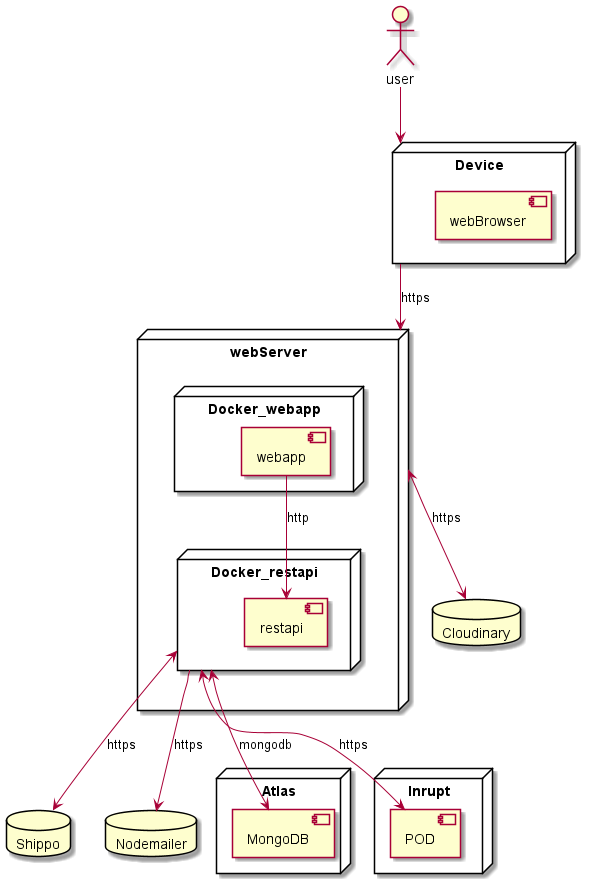
- Mapping of Building Blocks to Infrastructure
Elements | Description |
---|---|
Mobile or cumputer |
Are the devices from which users can access our application using a web browser |
Server |
Is in charge of storing and supporting our application |
MongoDB |
Is the database in which we will store the data of our application |
PODs |
Each user will have ono of this and personal data will be stored in it |
8. Cross-cutting Concepts
8.1. User interface
To create the user interface we will use the React library together with Typescript. React provides a great help when creating interfaces, as it is based on components, which are logical and self-contained pieces of code. By putting several components together, a user interface can be obtained.
8.2. Internationalization
We have decided that both the application and the documentation will be developed in English. This is because we consider that it can have a wider reach, since our application will not be exclusive to Spain, as it will be available to purchase from anywhere in the world.
8.3. Security
In terms of security, our application will follow SOLID principles and our users will use their own PODS to access it. The users will have a profile on our application where we will store only the needed information about them. The address is not going to be stored, every time the user orders a product we will ask him for his POD to get the address.
8.4. Build, Test, Deploy
We will follow a continuous integration process, in which after the creation of a new release using the GitHub Actions the application will be deployed.
The process to be followed will be:
-
Creation of a release (at stable points of the application).
-
When the release is generated, the GitHub Action will be executed doing the following:
-
First, the tests previously developed in restapi and webapp will be executed.
-
Once these tests are executed without any error, the acceptance tests will begin.
-
Once the acceptance tests are passed, the creation and uploading of the restapi and webapp docker images will begin.
-
At the end of the creation and uploading of the images, the application deployment on AWS will begin.
-
8.5. Persistence
For persistence we will use the MongoDB database together with the Mongoose ODM (Object Document Mapping), since it provides a lot of functionalities to work with MongoDB. We decided to use MongoDB as it is recommended for projects such as ours where we are developing an online store and it is very easy to integrate with Node.js, and since we are going to use the MERN stack (MongoDB, Express, React, Node), this comes in very handy when it comes to work.
8.6. Session handling
For the session we use inside the frontend the 'ReactSession' library to store the username and the token provided by our restapi api to identify it. This user information will be stored after a successful login.
8.7. Exception and error handling
For exception and error handling we will include several controls in the code. All user input will be validated to avoid errors because of what they may send us. In case an error is found, the user will be sent an error message that he can interpret, the error generated will not be sent directly to him.
8.8. Administration
To manage the application we will have at least one user with administrator role. This administrator will have different functionalities from those of a standard user.
Its functionalities will include the following:
-
Users management.
-
The administrator can delete users.
-
-
Products management.
-
Add new products.
-
Modify existing ones.
-
Delete products.
-
9. Design Decisions
This section shows the different design decisions presented in a table sorted by importance with their pros and cons.
Decision | Pros | Cons | Link |
---|---|---|---|
MongoDB |
Database that allows to modify data in a more flexible way |
No transactions and no atomicity |
|
MERN |
Allows the parts of the project to be unified in a simpler way |
Uses new technologies for team members |
|
mongoose |
API that facilitates the connection to MongoDB |
Never used |
|
AWS |
Platform that enables deployment of Docker images |
Problems related to downloading images from the virtual machine |
|
axios |
API allowing requests to be made to back-end from front-end |
Different response times for requests depending on the amount of data to be processed |
|
cloudinary |
Host that stores the product images for free |
We have never worked with an image processing API |
|
Cluster for tests |
Allows testing to be performed in a more secure manner as no application data is being worked on |
Increased number of connections and keys |
|
nodemailer |
It allows us to send emails from different hosts such as gmail, outlook… |
We have never worked with an email delivery API. |
|
react-toastify |
It allows you to create visual notifications in react in a simple way |
We have never worked with this library |
|
react-client-session |
A simple object to manage client session data in a React app. |
We have never worked with the user session on the web |
|
react-router-dom |
Library that allows to implement routes dynamically |
Learn how to use this library to create application routes and links to routes |
|
shippo |
API that automatically calculates shipping costs in a simple way |
Learn how to use the API and how to pass the address of a POD to it |
The rejected decisions are shown below.
Decision | Pros | Cons | Link |
---|---|---|---|
Heroku |
Allows projects to be deployed free of charge |
Difficulties in deploying multi-repository and Docker images |
10. Quality Requirements
10.1. Contents
This section shows the quality requirements tree with the description of each of them and the priority.
- Quality Tree
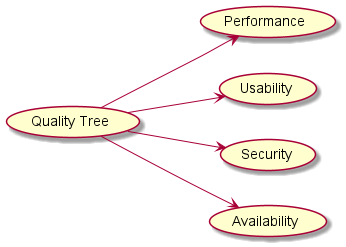
- Quality Scenarios
Identifier | Quality objectives | Scenarios | Priority | Difficulty |
---|---|---|---|---|
QG1-1 |
Security |
In Tech Zone the shipping address is obtained through the customer’s POD. |
High |
High |
QG1-2 |
Security |
The customer is the only person who knows what products he has in his cart. |
Medium |
Medium |
QG2-1 |
Usability |
During the login, registration and purchase process, notifications appear to help the customer in case of mistakes. |
Medium |
Low |
QG2-2 |
Usability |
The application is implemented in English so that it can be used by more people. |
Medium |
Low |
QG3 |
Availability |
The application is contained on an AWS machine that is accessible at all times while it is powered on. |
Medium |
Medium |
QG4 |
Performance |
We try to make our code as optimal as possible. We have a code duplicity of 4.7% that only appears in tests. |
Low |
High |
11. Risks and Technical Debts
Risk | Solutions |
---|---|
First time working with certain tecnologies |
Research on such technologies |
Lack of time regarding deliveries |
Set guidelines for a regular and stable progress |
Misunderstanding of the application requirements |
Clarify the requirements with the teacher |
Merge problems in git |
Set guidelines for a stable merge |
Options for editing products, canceling an order and banning users |
Implementing the frontend part of these options |
LogIn with SOLID |
Change the Solid API we use to one that allows users to login |
e2e testing in deployment |
Get the webapp to connect to restapi during the testing of these tests |
Continuous deployment |
Change the hosting plant, which would imply an economic cost |
12. Glossary
Term | Definition |
---|---|
SOLID |
Is a set of principles that make it easy for the developer to create a system that is easy to maintain and expand over time |
POD |
Are personal containers for data storage |
MERN |
Is a set of frameworks/technologies used for web application development consisting of MongoDB, React JS, Express JS and Node JS as its components |
MongoDB |
MongoDB is a document-oriented NoSQL database system |
mongoose |
An api that allows the connection between MongoDB and the application |
axios |
Simple HTTP client based on promises for the browser and node.js |
cloudinary |
Host for storing images in the cloud |
AWS - Amazon Web Services |
Collection of public cloud computing services that together form a cloud computing platform |
Heroku |
Platform that enables the deployment of applications |
API |
An API or application programming interface is a set of definitions and protocols used to design and integrate application software. |
Release |
Platform that enables the deployment of applications |
Continuous integration |
Continuous integration is a software engineering practice that consists of making automatic integrations of a project as often as possible in order to detect faults as soon as possible. |
Acceptance testing |
Acceptance testing is a test conducted to determine if the requirements of a specification or contract are met. |
ReactSession |
Store the data throughout the session and move the data between components. |
react-toastify |
Allows the creation of notifications for the application. |
react-router-dom |
Allows the creation of routes for the application. |
mui |
Library of React components. |
shippo |
API for calculating shipping costs from the USA with different parcel carriers. |
nodemailer |
Module for node.js that allows sending emails from different hosts such as gmail, outlook… |
About arc42
arc42, the Template for documentation of software and system architecture.
By Dr. Gernot Starke, Dr. Peter Hruschka and contributors.
Template Revision: 7.0 EN (based on asciidoc), January 2017
© We acknowledge that this document uses material from the arc 42 architecture template, http://www.arc42.de. Created by Dr. Peter Hruschka & Dr. Gernot Starke.