1. Introduction and Goals
1.1. Requirements Overview
1.2. Quality Goals
1.3. Stakeholders
2. Architecture Constraints
3. System Scope and Context
DeDe is an online shopping system where users choose the products they wish to purchase by adding one or more addresses to their User POD. The system is integrated with SOLID, where users manage their PODs and their data is under their control.
The system will only store user’s address, securing other type of personal data from being stored.
3.1. Business Context
Entity | Input | Output |
---|---|---|
User |
The user interact with the application using a computer or other device. |
The user output can be different, depending on the user inputs. |
User POD |
Each user has a POD, the user introduces data into the POD. |
The POD sends the required information to the application. |
Interface |
Recieves processed data |
Creates visual and interactive components for the user to see and use. |
API |
Recieves requests for processed data |
Processed data |
Database |
Recieves data |
Sends data |
3.2. Technical Context
The system uses a SOLID architecture, respecting user privacy, making personal information decentralized. For this we use user PODS, making users control their own information
Technology | Description |
---|---|
MongoDB |
Used as database of the WebApp. |
React |
Library to ease the programming of the application. |
TypeScript |
Used to program. |
4. Solution Strategy
4.1. Technology decisions
-
React: React makes it easy for us to program user interfaces using JavaScript.
-
Solid: SOLID gives the user the ability to choose what data they want to provide, and makes it easier for us to access it.
-
BootStrap: Bootstrap makes it easy for us to develop HTML and CSS for the web application
-
MongoDB: MongoDB is a non-relational database which allows us to not have to model all relationships.
4.2. How to achieve key quality objectives
Usability & Efficiently
The structure of the web would be similar to the ones already available on the market, this will provide a more easily to use application to the client. We will do some usability test with different user types during developing stages. All web and services will be optimized with the main aim of making queue waiting times faster.
Privacity & Security
We will ensure that the application is robust against the most common attacks, and we will ensure user privacy so that their data would not be leaked.
5. Building Block View
The building block view shows the static decomposition of the system into building blocks as well as their dependencies. It allows us to understand the system as a whole.
5.1. Whitebox Overall System
5.2. Level 1
5.3. Level 2
6. Runtime View
6.1. Add Rock to Shopping Cart
6.2. Log In / Register
The the runtime interactions inside the system to log or register are the following:
-
First the client enters the webpage.
-
Then clicks on login/register, it goes to the log in page.
-
The user is required to allow the the app to collect data from the POD.
-
If allowed, the POD gives the necessary data.
-
We look into our database to see if user exists, if exists brings back his data, if dont we store them.
-
Now the user is logged in and it goes back to the home page.
6.3. Purchase
The the runtime interactions inside the system purchase any item in our store are the following:
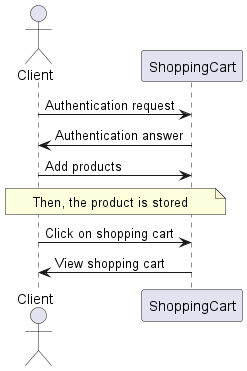
6.4. Delete Product from Shopping Cart.
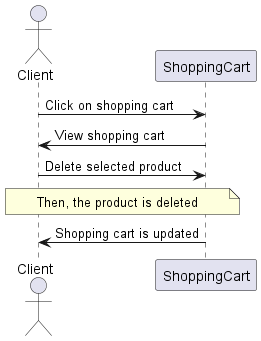
6.5. Login
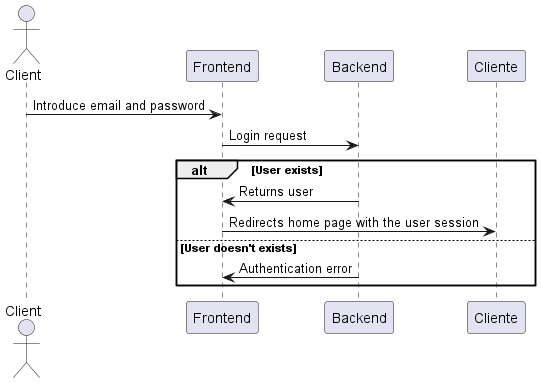
6.6. Register
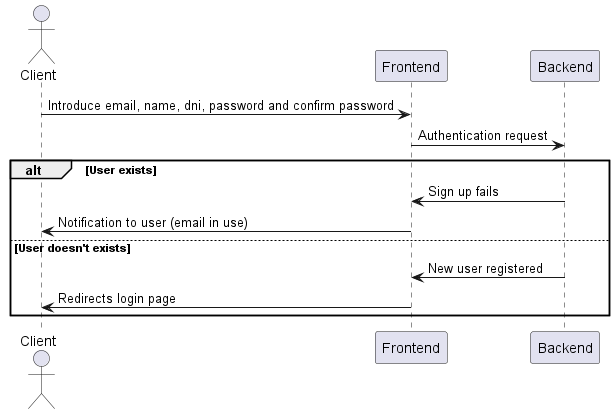
6.7. View User Orders
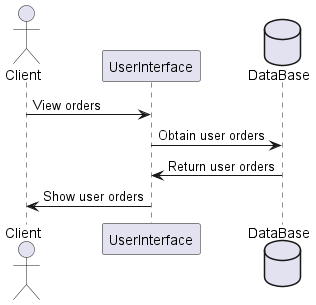
6.8. Filter Catalog
The the runtime interactions inside the system purchase any item in our store are the following:
-
First the client enters the webpage.
-
Then types the desired words to search for.
-
Clicks search.
-
The api processes the request and answers with the items.
-
The interface updates and shows the client their desired products.
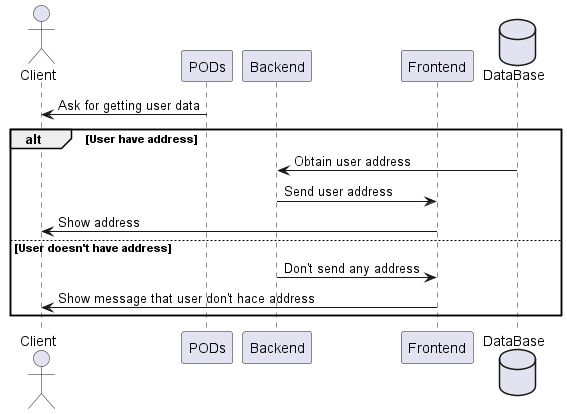
7. Deployment View
7.1. Infrastructure Level 1
- Motivation
-
By using this structure, you can see an overview of how the application will work in runtime
- Quality and/or Performance Features
-
Despite the fact that issues such as connection speed or performance depend mainly on other aspects such as the internet connection, we have tried our best to create a clean code that achieves the best performance for the user. With this, the intention is to achieve a good experience for the user in the use of the application.
- Mapping of Building Blocks to Infrastructure
Node | Description |
---|---|
Browser |
The method with which the user will connect to the application |
Ubuntu EC2 |
The virtual machine in which the application will be deployed |
Webapp |
The layer of views that the users will be able to see |
RestApi |
The layer of logic with which the application will connect |
USER POD |
The address of the user will be obtained from his POD |
ATLAS |
The provider that stores the database in mongodb |
DB MongoDB |
The database of the application with which the restapi will interact |
Cloudinary |
The API in which the images of the application’s products are stored |
8. Cross-cutting Concepts
8.1. Domain model
The address of the clients will be stored in their pods, being requested by the server to calculate the shipping costs. Once this process is done, the server will send the customer the final costs of their purchase.
8.2. Security and Safety
The app will provide users with a safe and secure environment. To carry this out the application will be developed using technologies such as SOLID taking into account its recommendations for use on issues related to data storage using pods. Another aspect is the use of protocols with the HTPPS for the connection with our application.
8.3. Usability
One of the important aspects of our application is that it can be used easily by any type of user. For this, it will be sought that the application has the least number of technical requirements in its use.
8.4. Accessibility
The color palette used in the application will be chosen taking into account that it is accessible to people with some type of color blindness.
9. Design Decisions
Decision | Reason |
---|---|
MogoDB |
This type of database is according to the requirements of the application. |
Visual Studio Code |
It will be the development enviroment used by all members of the group. One of its most favorable points is the possibility of adding a wide variety of extensions that will allow the project to be carried out in a simple way |
Cloudinary |
In this platform the images of the application will be stored, making it not necessary to save them locally |
Node Geocoder |
By using this library we get the coordinates from the user address stored in your POD |
Openstreetmap |
This will be the provider that we will use in the Node Geoder api, to obtain the coordinates of the user’s address |
10. Quality Requirements
10.1. Quality Tree
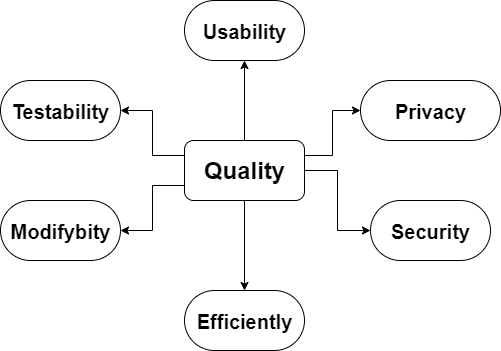
10.2. Quality Scenarios
ID | Quality attribute | Scenario | Priority User/Dev |
---|---|---|---|
U.1 |
Usability |
The application must be intuitive and easy to use bu most users |
High/High |
P.1 |
Privacity |
The user will have the power to choose the (non-essential) information that the application shows or has |
High/High |
S.1 |
Security |
The application should offer a certain security and integrity against attacks |
High/Medium |
M.1 |
Modifiability |
The application code should be easily extensible to add new functionality easily |
Low/High |
E.1 |
Efficiency |
The application must be optimized to minimize waiting times for services |
High/Medium |
T.1 |
Testability |
The application code should be easily testable, to facilitate testing to realiease a robust final product |
Low/High |
10.3. Risks and Technical Debts
10.4. At the moment
Risk |
Trade off |
Low knowledge of the use of PODS |
We have had little time to learn how this technology works. Invest more time to improve the state of the web. |
Time. We are conditioned by a delivery date and other subjects |
We must optimize individual and group work as much as possible |
It is complicated to test the functionalities of the application |
Increased work to design tests that test all the functionalities of the application |
10.5. At the beginning
Risk |
Trade off |
The interface is unfriendly to users |
We will have to invest time to test and code the flows through the interfaces |
The payment data of the purchases can intervene |
slower payment transactions because the use of SOLID |
An attacker can access and modify the data of our application |
More time to choose a robust db for the application |
Waiting times for application processes are very long |
Increased work to design a code as efficient as possible |
11. Glossary
Term | Definition |
---|---|
Decentralized |
Controlled by several local offices or authorities rather than one single one |
React |
Open source Javascript library designed to create user interfaces |
Usability |
Usability is a measure of how well a specific user in a specific context can use a product/design to achieve a defined goal effectively and efficiently |
Arc42 |
Arc42 is a template for architecture communication and documentation. |
Building Block |
Package of functionality defined to meet the business needs across an organization. |
Whitebox Overall System |
An overall view of how the system would interact with other systems or users. |
Runtime View |
Describes the behavior and interaction of the system’s building blocks as runtime elements |
API |
Application Programming Interface, which is a software intermediary that allows two applications to talk to each other. |
Solid POD |
Specification that lets people store their data securely in decentralized data stores. |
MongoDB |
MongoDB is an open source NoSQL database management program. |
NoSQL |
NoSQL is an approach to database design that enables the storage and querying of data outside the traditional structures found in relational databases |
BootStrap |
Bootstrap is a free and open source front end development framework for the creation of websites and web apps |
TypeScript |
TypeScript is a programming language developed and maintained by Microsoft |
Visual Studio Code |
Visual Studio Code is a lightweight but powerful source code editor which runs on your desktop and is available for Windows, macOS and Linux |
Cloudinary |
Cloudinary is an api that transform images and videos to load faster with no visual degradation, automatically generate image and video variants, and deliver high quality responsive experiences to increase conversions. |
OpenStreetMap |
OpenStreetMap is a collaborative project to create free and editable maps. |
U.1 |
Identificator for Usability |
P.1 |
Identificator for Privacity |
S.1 |
Identificator for Security |
M.1 |
Identificator for Modifiability |
E.1 |
Identificator for Efficiency |
T.1 |
Identificator for Testability |
About arc42
arc42, the Template for documentation of software and system architecture.
By Dr. Gernot Starke, Dr. Peter Hruschka and contributors.
Template Revision: 7.0 EN (based on asciidoc), January 2017
© We acknowledge that this document uses material from the arc 42 architecture template, http://www.arc42.de. Created by Dr. Peter Hruschka & Dr. Gernot Starke.