1. Introduction and Goals
This document describes the work carried out in the Software Architecture course project. The project consists in the development of a decentralized chat. The objetive of this chat is to provide a service in which the messages are not stored in a server but in each one of the nodes (users) of the system. The idea behind is similar to the Peer-to-Peer (P2P) approach for sharing content over the Internet.
1.1. Requirements Overview
The main requirement is to provide a system for sending and recieving messages with any server storing this data. Therefore the system will be based on a decentralized architecture where the data storage take place locally in each node. General overview of high-level requirements:
-
Users can store their data related with their chats in their own storage Pods.
-
Through the chat, users will be able to share photos, videos or any other type of files with their contacts
-
When a user wants to chat with a friend, the friend will be notified by an alert
-
Users can create new chat groups adding new people to them
With this project we want to achieve a private and point to point communication, i.e. without going through a server, thus avoiding information leaks and security problems.
-
UC1. Add Friends
-
UC2. New Chat
-
REQ001. The user will be able to add friend to the chat tool
-
REQ002. The user will be able to start a chat with one or more Friends
-
REQ003. The user will be able to create new chat groups
-
-
UC3. Replay message
-
REQ004. The user will be able to answer to a message sent to him
-
-
UC4. Multimedia files to a message
-
REQ005. The user will be able to send multimedia files in an attachment
-
1.2. Quality Goals
Priority |
Quality |
Motivation |
1 |
Functionality |
Users will be able to communicate effectively with others |
2 |
Descentrality |
Avoid security issues, among others |
3 |
Usability |
We want it to be able to be used and understood by any user |
4 |
Maintanability |
We follow a model view controller with which we can revising and extending funcionalities it’s easier |
1.3. Stakeholders
The following table contains the persons who are specifically targeted by the application
Role/Name |
Contact |
Expectations |
Project sponsor |
SOLID |
Determine which is the best chat and which best meets the requirements |
Professor |
Jose Emilio Labra Gayo |
Proof that the requirements are met and follow the project evolution |
Development Team |
Team4B |
Develop the application that has been entrusted to us in the framework of the subject of architecture of the software |
2. Architecture Constraints
2.1. Introduction
There are some constraints that limit the way we can implement our application. These constraints are different in type and scope, some of them are technical,some are organizational and others are conventions. The following tables detail these constraints.
2.2. Tables
Constraint | Explanation |
---|---|
Decentralized architecture |
We will use SOLID technology so user data can’t be centralized on a server. User data is not included in the application, so each user must be the one who keeps their information and the information they are sent. According to SOLID, that information will be stored in a POD |
Constraint | Explanation |
---|---|
Use Git as version control system |
Code evolution is going to be controlled by Git, leading to a colaborative development, allowing the team to have different code versions and to know exactly who changed the code in each moment. |
Use GitHub as Configuration Management system |
Our project must be managed using GitHub so we can be well organized as a team know who did any change on the code and of course, GitHub makes easier the colaborative development. |
Time restriction |
A deadline date is set for completion of the project and there are some points in which project’s progress will be reviewed. The deadline for our team is May 3rd |
Budget |
Budget is not existant, so we have to limit ourselves to the use of free tools. |
Constraint | Explanation |
---|---|
Use of Arc42 template for documentation |
We must use this template to maintain an order and make sure than anyone who reads it can find what they are looking for, knowing the section in which it is located |
3. System Scope and Context
3.1. Business Context
There are different functions in the application.
Functions | Definition |
---|---|
Chat with friends |
Exchange messages with another user. |
Share files |
Send files like pictures, videos or other kinds of files to your friends. |
Create groups |
You can create a group of users for chatting with them like you do with a friend. |
Get notifications |
When some friend sends you a message. You will receive a notification. |
Store data |
This function needs connection to your PODs. It saves the data into your private POD when you send a message or a file. |
3.2. Technical Context
Our application is a decentralized web application, the data will be stored in the Pods of the users. Therefore our application must be connected to the Internet.
Users will communicate with each other through URLs, through the Internet, without the need for a central place to store the data.
Legend of diagram
Object |
Description |
DeChat |
Is the web application |
Internet |
Represents the communication will be made using internet with the HTTPS protocol. |
Pod |
This is a Solid Pod and it will contain all the data from the given user |
User |
Represents a user that is using the application |
SOLID |
Is the server in which the Solid pods are stored |
4. Solution Strategy
4.1. Introduction
In this part we will documentate a short summary and explanation of the decisions and solutions in our project like technology decisions, architectonical decisions or organizational decisions.
4.2. Decisions
Short explanation of the decisions of the project
-
Cucumber: it runs automated acceptance tests written in a behavior-driven development style
-
Javascript: Is a lenguage based on ECMASCRIPT, with it we can do applications in the web. We will use Javascript because in the project we will implementate a chat, as we know, and this language is the best and easiest for client applications.
-
MochaJs: is a JavaScript test framework for Node.js programs, featuring browser support, asynchronous testing, test coverage reports, and use of any assertion library.
-
Node.js: Is an open-source, cross-platform JavaScript run-time environment that executes JavaScript code outside of a browser.
-
Solid: Is a web decentralization project, just what we need to do the project.
-
Travis: is a hosted, distributed continuous integration service used to build and test software projects hosted at GitHub.
-
Turtle: is a syntax and file format for expressing data in the Resource Description Framework data model, we need it to store information on the PODs.
4.3. Solution Aproaches
Once we started to do the project of the chat, we took some direct solutions. We started to programming in Javascript based in the project of a Solid Chess. We will implement some integration test with Travis to take advantage of the continuous integration service using Mocha and his assertion library to help us to prove and improve the code. We can connect to the pod and we can send messages with Javascript using JQuery. The project is developed in Node.js which is better for us than use Angular for all the problems Angular can have for us while Node is not difficult because we know some JavaScript. We added some TDD testing with cucumber too which is important to prove that our code is working. Once we can communicate with other people, that is the main purpose of this application, we added images to the communication and chat groups to communicate with more than one person. We added an option to add friends. Finally we improve all of the interface of the application to make it easier to use, for this we improve the CSS and HTML files.
5. Building Block View
5.1. Whitebox Overall System
DeChat is a descentralized chat created with SOLID. SOLID is a project led by Tim Berners-Lee wich aims to change the way Web Apps work today. To make this posible, as a first part of the project, SOLID has created SOLID Pods. In a future stage of the development these pods will not be neccesary.
After this introduction, let’s talk about our project and how all will work. The App will have three main elements: pods, users and DeChat App. These parts will comunicate with each other.
-
User: users will use DeChat App to comunicate to other users.
-
DeChat App: the app will provide all the funcionality to users and will comunicate with SOLID Pods.
-
SOLID Pods: they will work as an intermediary between the diferent apps of users.
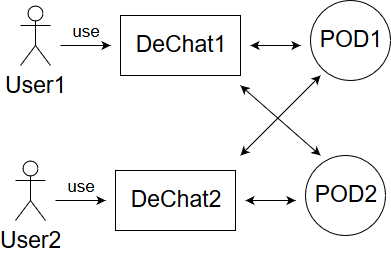
5.2. Level 2
Our chat is built using three main blocks:
- User Interface
-
An single HTML file to present all the application. To control what we want to display every moment it’s used JavaScript, and specially jQuery library.
- Logic
-
This block is used to connect PODs administration with User Interface.
- Data Storing
-
In this project we are using SOLID PODs. The main reason: each user is owner of his own data. This block will be written and readed by logic, and after that present the data.
5.3. Level 3
- index
-
the main module in the system is index.js. It is used to control every action user does in the page, and call other modules and functions.
- core
-
is used to administrate the communications. This module uses another more specific modules like the communication of groups or personal (private). They will connect with PODs.
- private communication
-
Responsible for communications between users, sending and retrieval of messages.
- group communication
-
Responsible for communications between users that share a group, sending and retrieval of messages.
- encrypt
-
Codes from plain text to base64 and vice versa.
- notification manager
-
Manages the occurrence of notifications when a message is received or a group is created
- personal
-
used to load data from users.
- messages
-
A simple class with different attributes to store messages
6. Runtime View
6.1. Fitst Scenario: Login
Although basic is one of the most important scenarios because if a user is not logged in can not do anything.
-
User opens the app in his browser. User could be logged or not.
-
If the user is logged in, he will be able to start using the app.
-
If he is not logged in, he can click the login button. A popup in which the user must enter his Solid credentials (from solid community, inrupt, his WebID). In case the user does not have an account he will be able to create one.
-
-
Once the user is logged in one way or another, the app will allow him to start a chat, continue a indivudual or group chat and create new groups, as friends and groups are loaded as soon as the sing in process is done.
6.2. Second Scenario: Send a message
The main functionality of the application is to send messages. Here we show how to di it.
-
We assume that the user is logged in. If not he won’t be able to chat.
-
The user clicks the New Chat button. A list of his friends in solid and groups he is part of is shown in a ComboBox.
-
The user looks for his friend or group and selects an option.
-
If the individual or group conversation has already started, messages, images and emotes will be loaded from the POD to the panel by the communication module(Group or individual). If on the other hand the message is the first of the conversation the panel will appear empty.
-
The message is written in the text area and when the user clicks the button or enter key, it is sent.
-
If the conversation is individual the message will be stored in user´s own POD and will give the other user permission to read it.
-
If it is a gropup conversation, the message will be stored in group creator’s POD.
-
-
The user or users in the chat will be able to load this message immediately if they are on the chat. If not, the next time they sign up to the app, the message will be loaded.
6.3. Third Scenario: Create a group
-
One more time We assume that the user is logged in.
-
The user clicks the Create Group button. A list of his friends in solid are loaded from his account and a TextBox is displayed to enter a group name.
-
He enters the name and select the checkBoxes of friends he want to be in the grop.
-
Finally the user clicks the create button.
-
From the main view of the app, the user will be able to select new chat. The name of the newly created group will appear in the combobox mentioned above.
7. Deployment View
Our chat deChat is a client side application that runs in user’s browser to connect to a Solid POD to chat with our friends, so to use it we only need a computer and an Internet connection, we developed this application in Firefox and Chrome, so those are the browsers we recommend to use.
7.1. Infrastructure Level 1
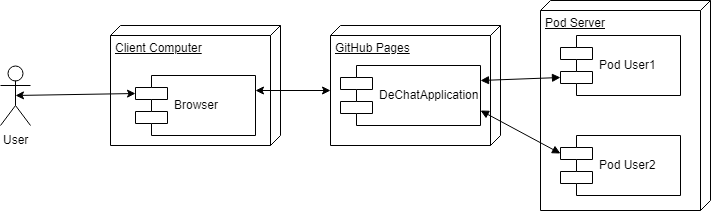
We can see a first node which is the node of the computer of the user, he connect to the browser with Internet. The second node is the application, we need a server to connect to the chat. Finally we connect to the last node, this node is SOLID Server who provides us user’s POD and the receiver or receivers POD, we connect to user’s pod to store the message and we send it to the receiver or receivers.
The distribution of our system was the same in all scenarios because we only need a computer with internet, we could test it in home and in the university the same way. Since the start, we knew this was a browser application and SOLID was our system to storage information of our communication, so we implementate the chat for a browser like Firefox, so we obviously need a computer with internet connection and the browser. Any computer can use dechat application. After connecting to the chat in the browser we use internet to connect to the SOLID Server and now we can send messages to another person.
8. Cross-cutting Concepts
This section describes regulation and solution ideas that are relevant in multiple parts of our system.
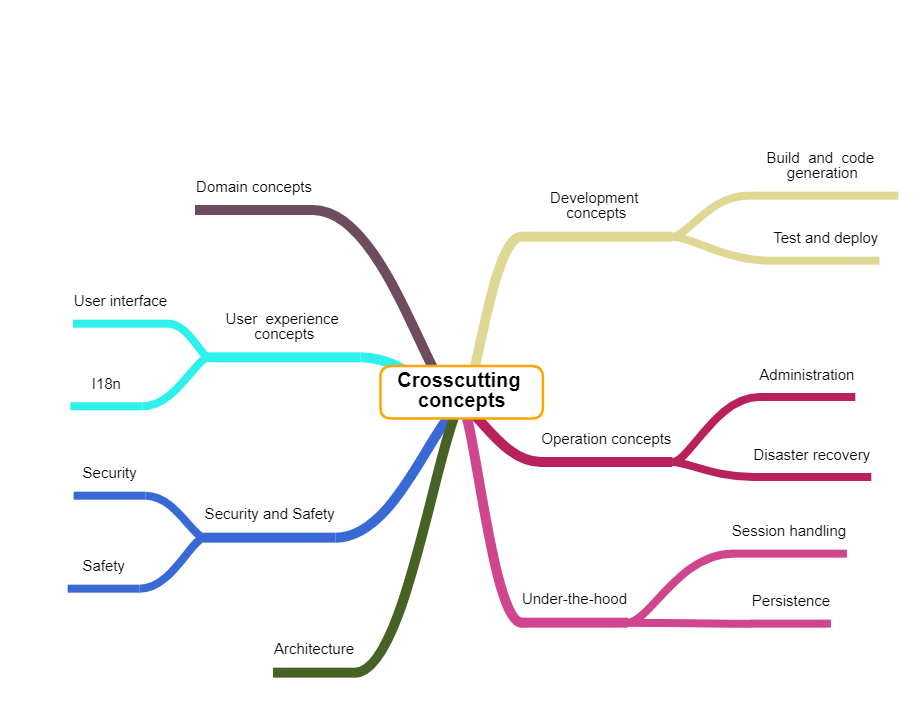
8.1. Domain concepts
-
Domain models We use a domain where we have an index file which depends of libraries, then we have various folders when we store testing and documentation.
8.2. User Experience concepts
-
User interface As user interface we can understand the interface of the application, in this case the interface is a normal chat interface like other chats applications.
-
Internationalization We did the application in English and for the moment we will not add internationalization to other lenguages.
8.3. Safety and security concepts
-
Security and safety The security and safety in the project is key because this application is based in Solid which is decentralized and safe. We store the information we send in the PODs to avoid problems in the security.
8.4. Architecture and design patterns
-
P2P Architecture is a commonly used computer networking architecture in which each workstation, or node, has the same capabilities and responsibilities.
8.5. “Under-the-hood” concepts
-
Session handling We log in with our user of SOLID in the application, once we are into the service we can communicate with the server to interact with other users.
-
Persistence to save our information, we store it on PODs on SOLID, so we don’t use information stored in databases.
-
Communication and integration in this chat we use asynchronous methods. We are working on the integration in another sites.
8.6. Development concepts
-
Build and code generation The build and code generation in our project is done in javascript with Node.js. We must have instaled Node in our computer to build the project.
-
Test and deploy For testing we use cucumber for acceptance testing and we use BDD. For asynchronous testing we use mocha. To deploy the project we must have Node installed.
8.7. Operational concepts
-
Administration For the administration of this project we work as a team so we administrate it equally.
-
Disaster recovery We have this project in GitHub so if something happened to the project locally, we have a copy there.
9. Design Decisions
At this stage of the project few design decisions have been taken.
We will follow a P2P architecture without a dedicated database, all users information will be stores in their PODs.
Property |
Decision |
Explanation |
Architecture |
Peer-to-Peer (P2P) |
It is mandatory in a decentralized Solid app |
Framework |
None |
We started the project with Angular in mind, but we rejected it after see its complexity, the lack of previous experience by all of us and after see other groups complaining about the same decision |
Run-time environment |
Node.js |
Although Node is not a framework, we chose this technology after abandon Angular because of its simplicity that allows us to deploy a 0.1 version fast. |
Database |
None |
There is no need for a database, each chat user will store their data and messages in their personal POD |
10. Quality Requirements
10.1. Quality Tree
The project has some important quality requirements. They will be presented in a diagram and specified in a table
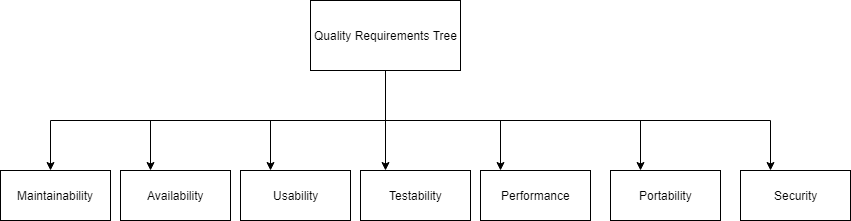
10.2. Quality Scenarios
Here you can see a little more in depth the main quality scenarios that have already been seen before in the quality tree.
Reference |
Quality Requirement |
Scenario |
Priority |
1 |
Availability |
The system must be accessible most of the time.We set a limit at 95% of the time |
Medium |
2 |
Maintainability |
A group of 4 developers should introduce a functionality, such as sending stickers within 2 days. |
High |
3 |
Testability |
Good software must be testable. Code coverage should be high. BDD and TDD tests must be done |
High |
4 |
Usability |
Everyone should use the app whithout problems regardless of their capabilities |
High |
5 |
Performance |
Messages should be sent fast. The user should not perceive waiting time |
Medium |
6 |
Portability |
The application should be able to be used on any computer with any browser |
Medium |
8 |
Security |
Messages must be stored encrypted |
Medium |
9 |
Security |
You will not be able to read chats that you are not a user involved in |
High |
10 |
Availability |
A user will be able to access his messages at any time |
High |
11. Risks and Technical Debts
This page contains a list of all the risks we can encounter during the project as well as measures to try to minimize and solve them.
11.1. Risks:
-
Solid Is a completely new technology to the group and it might take us time until we get used to its functionality, the concept of POD is not something we are familiariced.
-
Javascript lthough we’ve used it in the past, it’s not our go-to programming language.
-
Teamates none of us have worked with this many people before and some of us didn’t know each other before. This is a problem we have to do this as a team.
-
English is not our first language and it can lead to some errors in the documentation.
-
Turtle we didn’t know this syntax which was a difficult thing to front.
-
Asynchronous Programming this is a problem when we try to do testing and functions, because is something we never worked on it.
11.2. Measures to solve them:
-
Try to learn as much as possible about Solid and Javascript before starting to work on the actual code, and keep researching and learning during the whole project.
-
Increasing the communication in the group so everyone know what everyone is doing and has to do.
-
Do some research on Turtle syntax and Asynchronous programming and search examples to work on it.
12. Glossary
Term | Definition |
---|---|
Arc42 |
Template for architecture communication and documentation. It divides the documentation of the architecture in diferentiated sections |
BDD |
Behavior-driven development combines the general techniques and principles of TDD with ideas from domain-driven design and object-oriented analysis and design to provide software development and management teams with shared tools and a shared process to collaborate on software development. |
Chai |
Behavior-driven development assertion library compatible with Mocha and other JavaScript test frameworks. |
CSS |
Is a style sheet language used for describing the presentation of a document written in a markup language like HTML. |
Decentralized computing |
Decentralized computing is the allocation of resources, both hardware and software, to each individual workstation, or office location. In contrast, centralized computing exists when the majority of functions are carried out, or obtained from a remote centralized location. |
ECMASCRIPT |
Trademarked scripting-language specification standarized by ECMA International. It was created to standardize JavaScript. |
HTML |
Hyper Text Markup Language (HTML) describes the structure of Web pages using markup. |
Integration testing |
Is the phase in software testing in which individual software modules are combined and tested as a group. |
JQuery |
Is a JavaScript library designed to simplify HTML DOM tree traversal and manipulation, as well as event handling, CSS animation, and Ajax. |
MEAN |
Set of 4 open source tools used for web development. Its components are MongoDB, Express.js, Angular.js and Node.js. |
Mocha |
JavaScript framework to run asynchronous tests on Node.js |
MVC architecture |
The MVC or Model-View-Controller is a software architecture pattern that, using 3 components (Views, Models and Controllers) separates the logic of the application from the logic of the view in an application. |
POD |
Personal online data store . It allows users to save their information and the information they are sent. A POD can store photos, text, contacts or calendar events and many other things |
POD Provider |
A person or company that has one or more solid pod servers and uses them to host consumer data. |
POD Server |
Server that allows the application store POD information on it. |
SOLID |
Technology whose goal is to change the way applications interact with user data, giving more decision-making power to users as they are able to decide where their data is stored or who can read it or write it. |
SOLID file client |
Library that provides a simple interface for logging in and out of a Solid data store, maintaining a persistent session, and for managing files and folders. |
Stakeholder |
Person with an interest or concern in something, in the area we are working on, the decentralized chat. |
TDD |
Software development process that relies on the repetition of a very short development cycle: requirements are turned into very specific test cases, then the software is improved to pass the new tests, only. |
Turtle |
is a syntax and file format for expressing data in the Resource Description Framework (RDF) data model. |
Type-Script |
An open-source programming language, is a strict syntactical superset of JavaScript, and adds optional static typing to the language. |
User |
A person who uses a particular application or computer system. |
About arc42
arc42, the Template for documentation of software and system architecture.
By Dr. Gernot Starke, Dr. Peter Hruschka and contributors.
Template Revision: 7.0 EN (based on asciidoc), January 2017
© We acknowledge that this document uses material from the arc 42 architecture template, http://www.arc42.de. Created by Dr. Peter Hruschka & Dr. Gernot Starke.